NPM Notes, Tips & Tricks
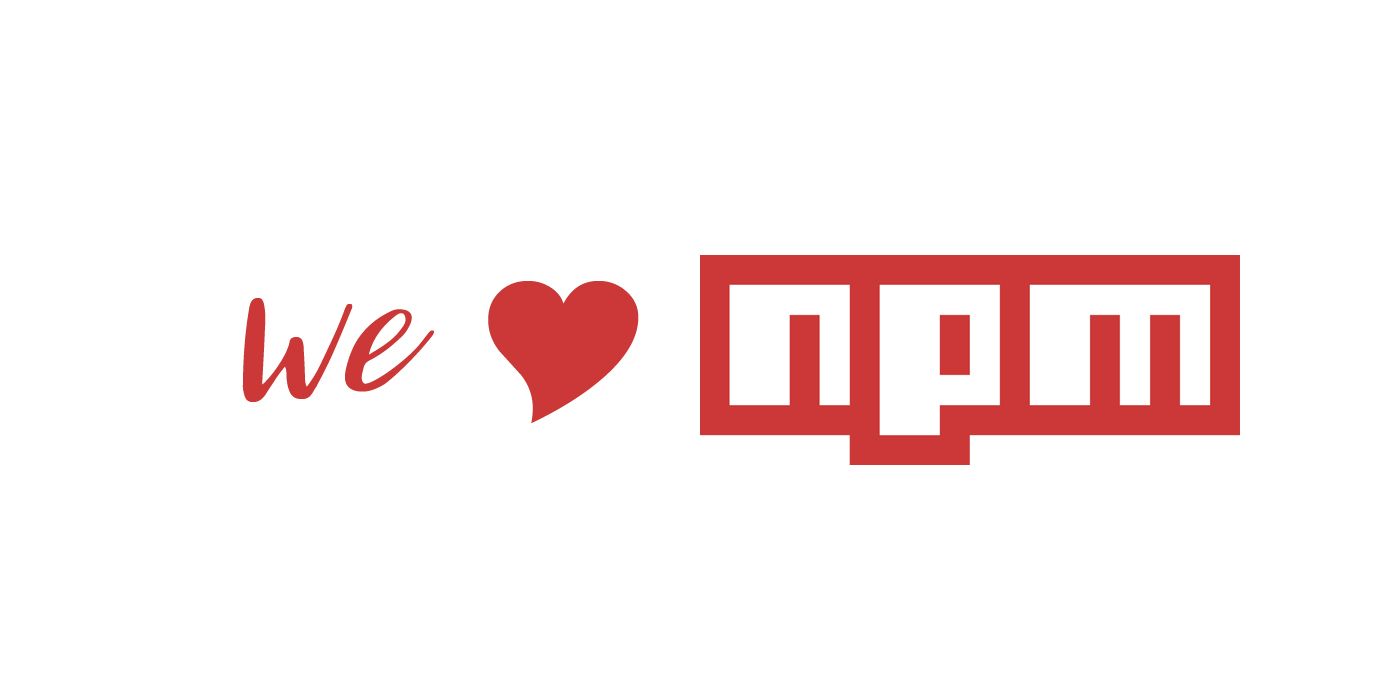
Just a fun trivia to begin with!
NPM downloads yesterday (23 Mar, 2017): 369 million.
NuGet downloads in the last week: 55 million.
Don't we love the Node Package Manager - NPM & Open Source Software in general?
This article focuses on some of the cool tips and tricks related to NPM and I hope you find it helpful.
Package vs. Module
A Package is a just a directory with one more files in it along with a package.json
file that contains metadata about the package. A Module on the other hand... is just a simple file. Every file is treated as a separate module and in Node.js file:module is a 1:1 proposition.
Initialize > Install > Start
A typical flow for a Node.js application when you start from scratch is:
- Initialize using
npm init
which in turn creates apackage.json
file. - Install dependencies using
npm install
ornpm install --save
ornpm install --save-dev
.--save
switch will save the information about the package in dependencies section, whereas--save-dev
switch saves it under devDependencies section.
Can you guess which switches have been used below?
[npm-demo] $ cat package.json
{
"name": "npm-demo",
"version": "1.0.0",
"summary": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC",
"dependencies": {
"express": "^4.15.2",
"lodash": "^4.17.4"
},
"devDependencies": {
"underscore": "^1.8.3"
}
}
Clone > Install > Start
Usually, you would clone a repository, install the dependencies and do an npm start
like so:
- Step 1:
git clone https://github.com/TryGhost/Ghost.git
(switch to the folder which has package.json) - Step 2:
npm install
to install dependencies. - Step 3:
npm start
to start the application.
This is pretty cool, no?
start, test, init
npm start
: Starts your application based on scripts
section in the package.json
file.
npm test
: Execute your tests
.
npm init
: Execute your initialization code.
Notice the presence of start
, test
and init
in package.json
for
Ghost.
"scripts": {
"start": "node index",
"test": "grunt validate --verbose",
"init": "npm install -g knex-migrator ember-cli grunt-cli && npm install && grunt init"
}
Getting Help
npm -h
ornpm -help
: Shows brief help.npm -h install
: Shows brief help about a switch.npm help install
: Shows detailed help about a switch.npm help-search remove
: Searches for a keyword, in this case:remove
, assuming you want to know which commands help you remove.
[Ghost] $ npm help-search remove
Top hits for "remove"
————————————————————————————————————————————————————————————————————————————————
npm help config remove:6
npm help unpublish remove:5
npm help uninstall remove:5
npm help index remove:4
npm help prune remove:4
npm help removing-npm remove:2
npm help bundle remove:2
npm help publish remove:1
npm help team remove:1
npm help owner remove:1
npm help package.json remove:1
npm help coding-style remove:1
npm help install remove:1
npm help disputes remove:1
npm help dist-tag remove:1
npm help access remove:1
————————————————————————————————————————————————————————————————————————————————
(run with -l or --long to see more context)
Now you can check the different commands like prune
, unpublish
, removing-npm
, etc.
NPM Default Configuration
npm config list
: List all configuration defaults.npm config edit --global
: View all the NPM configuration defaults.
;;;;
; npm userconfig file
; this is a simple ini-formatted file
; lines that start with semi-colons are comments.
; read `npm help config` for help on the various options
;;;;
//registry.npmjs.org/:_authToken=********
;;;;
; all options with default values
;;;;
; access=null
; always-auth=false
; also=null
; auth-type=legacy
; bin-links=true
; browser=null
; ca=null
; cafile=undefined
; cache=/Users/ rahul sonisoni/.npm
; cache-lock-stale=60000
; cache-lock-retries=10
; cache-lock-wait=10000
; cache-max=null
; cache-min=10
; cert=null
; color=true
; depth=null
; summary=true
; dev=false
; dry-run=false
; editor=vi
; engine-strict=false
; force=false
; fetch-retries=2
; fetch-retry-factor=10
; fetch-retry-mintimeout=10000
; fetch-retry-maxtimeout=60000
; git=git
; git-tag-version=true
; global=false
; globalconfig=/usr/local/etc/npmrc
; global-style=false
; group=20
; heading=npm
; if-present=false
; ignore-scripts=false
; init-module=/Users/ rahul sonisoni/.npm-init.js
; init-author-name=
; init-author-email=
; init-author-url=
; init-version=1.0.0
; init-license=ISC
; json=false
; key=null
; legacy-bundling=false
; link=false
; local-address=undefined
; loglevel=warn
; logs-max=10
; long=false
; maxsockets=50
; message=%s
; metrics-registry=null
; node-version=4.2.3
; onload-script=null
; only=null
; optional=true
; parseable=false
; prefix=/usr/local
; production=false
; progress=true
; proprietary-attribs=true
; proxy=null
; https-proxy=null
; user-agent=npm/{npm-version} node/{node-version} {platform} {arch}
; rebuild-bundle=true
; registry=https://registry.npmjs.org/
; rollback=true
; save=false
; save-bundle=false
; save-dev=false
; save-exact=false
; save-optional=false
; save-prefix=^
; scope=
; scripts-prepend-node-path=warn-only
; searchopts=
; searchexclude=null
; searchlimit=20
; searchstaleness=900
; send-metrics=false
; shell=/bin/bash
; shrinkwrap=true
; sign-git-tag=false
; sso-poll-frequency=500
; sso-type=oauth
; strict-ssl=true
; tag=latest
; tag-version-prefix=v
; tmp=/var/folders/w7/rcgmhk4j2sv1sxq5_dv1tr1c000ddd/T
; unicode=true
; unsafe-perm=true
; usage=false
; user=0
; userconfig=/Users/ rahul sonisoni/.npmrc
; umask=18
; version=false
; versions=false
; viewer=man
; _exit=true
; globalignorefile=/usr/local/etc/npmignore
npm config get userconfig
: Gives you the path of the.npmrc file
which contains all the configuration information.npm config get maxsockets
: Gives you details aboutmaxsockets
configuration inside of the.npmrc
file. You can similarly get any key, or set it usingnpm config set
command.
NPM Install
npm install lodash
: Install the package calledlodash
.npm install lodash -g
: Install the package globally.npm list
: List the entire dependency tree of all the installed packages.npm list -depth 1
: Shows only the list of dependencies till depth 1.npm list -depth 0 -dev
: Shows only root level development dependencies.npm list -g -depth 0
: Shows the global dependencies.npm list -g -depth 0 -long
: Shows a lot more details about the installed dependencies.
Note:
- You might need to use
sudo
to install with a-g
switch. - You can use
-D
instead of--save-dev
switch and-S
for--save
.
NPM Update
npm update
: Updates all the dependencies.npm update --dev -S
: Updates all the dev dependencies.npm update --prod -S
: Updates all the prod dependencies.npm update -g
: Updates all the global dependencies.npm install package@x.x.x
- Install a specific version.
NPM Uninstall
npm uninstall package --save
andnpm uninstall package --save-dev
uninstalls the package from your folder and updates thepackage.json
file.npm uninstall package -g
uninstalls a global package.npm cache clean
: Cleans the npm cache.
I hope this helps. Do you know of a command that you use often or other tips that can help? Please post it in the comments section.
What next?
Stay tuned for upcoming articles. You may contact us for your software and consultancy requirements.