Mastering PowerShell Loops and Conditional Statements: A Comprehensive Guide
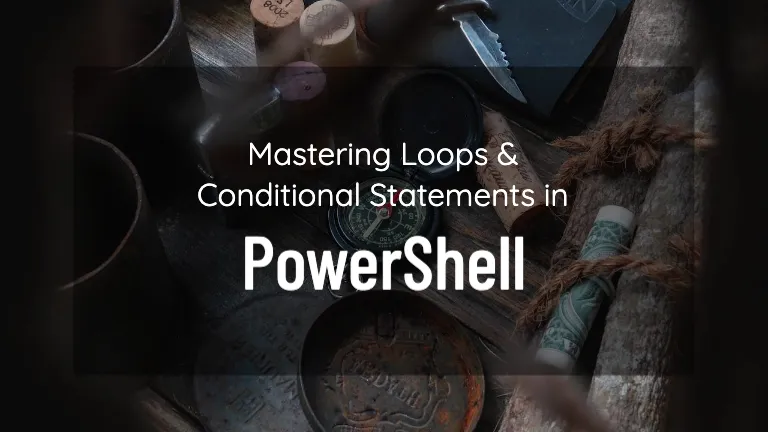
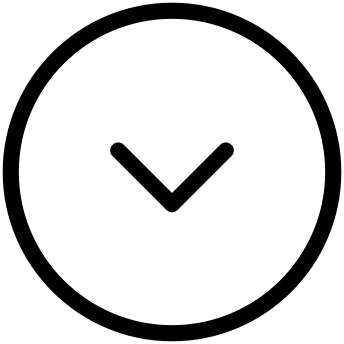
Mastering Loops and Conditional Statements in PowerShell
PowerShell is a powerful scripting language that allows users to automate administrative tasks, manipulate data, and interact with systems efficiently. One of the core components of scripting in PowerShell is the ability to control the flow of logic using loops and conditional statements. In this blog post, we’ll explore different types of loops and conditional statements in PowerShell, providing examples to help you understand how to use them effectively.
Conditional Statements
Conditional statements allow you to execute different blocks of code based on certain conditions. Here are the main types of conditional statements in PowerShell:
If-Else Statements
The if
statement evaluates a condition and executes a block of code if the condition is true. You can use elseif
and else
to handle additional cases.
$number = Get-Random -Minimum 1 -Maximum 5
if ($number -eq 1) {
Write-Host "Having 1 girlfriend is ok"
} elseif ($number -eq 2) {
Write-Host "Having 2 is better than 1, probably... but ensure they don't bump into each other 😉"
} else {
Write-Host "How much time do you have man, slow down 😅"
}
Switch Statements
The switch
statement compares a variable against a set of values and executes the matching block of code.
$food = Get-Random -Minimum 1 -Maximum 4
switch ($food) {
1 { Write-Output "Biryani: classic favorite for every occasion!" }
2 { Write-Output "Butter Chicken: Rich and delicious comfort food." }
3 { Write-Output "Samosa: Perfect for a quick snack." }
default { Write-Output "No food for you!" }
}
Ternary Operator
Recently, PowerShell introduced a ternary-like operator for simple conditional assignments.
$age = Get-Random -Minimum 16 -Maximum 25
$message = $age -ge 18 ? "You are eligible to vote in India" : "You are not eligible to vote, yet!"
Write-Host $message
Loops
Loops are used to execute a block of code repeatedly. PowerShell supports several types of loops:
For Loop
A for
loop is ideal for executing a block of code a specific number of times.
Write-Host "Counting whole numbers till 5..."
for ($number = 0; $number -le 5; $number++) {
Write-Host "Number: $number"
}
#output
Counting whole numbers till 5...
Number: 0
Number: 1
Number: 2
Number: 3
Number: 4
Number: 5
Foreach Loop
The foreach
loop iterates over each item in a collection.
Write-Host "Counting natural numbers till 5..."
$numbers = 1..5
foreach ($number in $numbers) {
Write-Host "Number: $number"
$number++
}
#output
Counting natural numbers till 5...
Number: 1
Number: 2
Number: 3
Number: 4
Number: 5
While Loop
A while
loop continues to execute as long as its condition evaluates to true.
Write-Host "Counting natural numbers till 5... (run till the condition becomes false)"
$number = 1
while ($number -le 5) {
Write-Host "Number: $number"
$number++
}
#output
Counting natural numbers till 5... (run till the condition becomes false)
Number: 1
Number: 2
Number: 3
Number: 4
Number: 5
Do-While Loop
The do-while
loop guarantees at least one execution of the block before evaluating the condition.
Write-Host "Counting natural numbers till 5... (run at least once, and till the condition becomes false)"
$number = 1
do {
Write-Host "Number: $number"
$number++
} while ($number -le 5)
#output
Counting natural numbers till 5... (run at least once, and till the condition becomes false)
Number: 1
Number: 2
Number: 3
Number: 4
Number: 5
Do-Until Loop
The do-until
loop is similar to do-while
but continues until the condition becomes true.
Write-Host "Counting natural numbers till 5... (until the condition becomes true)"
$number = 1
do {
Write-Host "Number: $number"
$number++
} until ($number -eq 5)
# notice that the output only prints till 4
Counting natural numbers till 5... (until the condition becomes true)
Number: 1
Number: 2
Number: 3
Number: 4
Break and Continue Statements
PowerShell provides break
and continue
statements to control loop execution:
Break Statement
Exits the current loop immediately.
foreach ($number in 1..100) {
if ($number -eq 5) {
Write-host "Breaking out of loop at $number"
break
}
Write-Host "Number: $number"
}
#output
Number: 1
Number: 2
Number: 3
Number: 4
Breaking out of loop at 5
Continue Statement
Skips the current iteration and proceeds with the next one.
foreach ($number in 1..10) {
# skip this iteration if the number is an even number
if ($number % 2 -eq 0) { continue }
Write-Host "Odd Number: $number"
}
#output
Odd Number: 1
Odd Number: 3
Odd Number: 5
Odd Number: 7
Odd Number: 9
Infinite Loops and Exit Conditions
Be cautious with loops that have conditions that may never evaluate to false, creating an infinite loop. Use break
or proper exit conditions to handle such scenarios.
while ($true) {
Write-Host "Breaking out of an infinite loop."
break # Exits the loop to avoid infinite execution.
}
Combining Loops and Conditionals
Combining loops and conditionals lets you solve complex problems. Here’s an example that finds prime numbers within a range:
$range = 2..50
$factor = 1
foreach ($number in $range) {
$isPrime = $true
for ($divisor = 2; $divisor -lt $number; $divisor++) {
if ($number % $divisor -eq 0) {
$isPrime = $false
$factor = $divisor
break
}
}
if ($isPrime) {
Write-Host "$number is a prime number."
}
}
#output
2 is a prime number.
3 is a prime number.
5 is a prime number.
7 is a prime number.
11 is a prime number.
13 is a prime number.
17 is a prime number.
19 is a prime number.
23 is a prime number.
29 is a prime number.
31 is a prime number.
37 is a prime number.
41 is a prime number.
43 is a prime number.
47 is a prime number.
Conclusion
Understanding loops and conditional statements in PowerShell is essential for effective scripting and automation. By mastering these constructs, you can handle everything from simple tasks to complex workflows efficiently. Start experimenting with these examples, and soon, you’ll find yourself writing powerful PowerShell scripts with ease!
Hope this helps! 👋