PowerShell Shorthand Notations: A quick reference guide
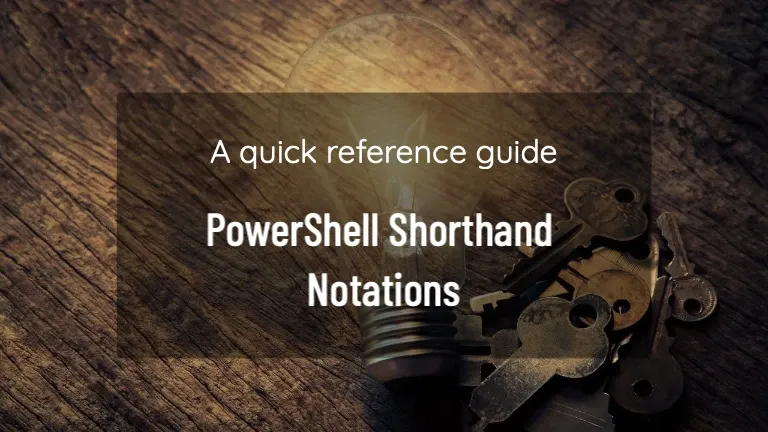
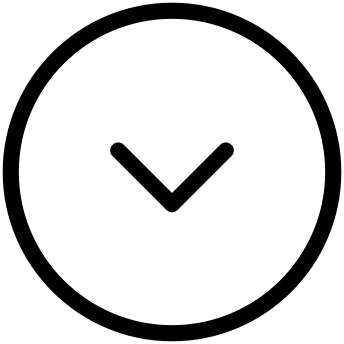
PowerShell is packed with shorthand notations that make it concise and powerful for scripting and automation. Hereβs a quick dive into the most commonly used ones to help you work faster and smarter.
$ - Variable Declaration and Access
$name = "PowerShell"
Write-Output $name
Use it for storing and accessing values in scripts.
$_ - Current Object in the Pipeline
Get-Process | Where-Object { $_.CPU -gt 100 }
Use it for Filtering, transforming, or processing objects in pipelines. If you are coming from programming background, think of $_
as this
.
% - Alias for ForEach-Object
Get-Process | % { $_.Name }
Use it for looping through the items.
? - Alias for Where-Object
Get-Process | ? { $_.Name -like '*system*' }
Use it to filtering data based on conditions.
@ - Array and Hashtable Notation
# Array
$array = @(1, 2, 3)
# Hashtable
$hash = @{ Key = "Value"; AnotherKey = 42 }
Use it to group data structures. It defines arrays or data structures.
@@ - Splatting
#hashtable
$params = @{ Name = "MyFile.txt"; Path = "/my/path" }
New-Item @params
#array
$params = @("MyFile.txt", "/my/path" }
New-Item @params
Use it to simplify parameter heavy commands. It passes a collection of parameters to a command.
& - Call Operator
& "/path/to/script.ps1"
It is particularly useful when you want to execute a command whose name is stored in a variable or when constructing commands programmatically.
. - Dot Sourcing
. "/path/to/script.ps1"
Use it to retaining variables and functions in the current session.
:: - Static Member Access
[Math]::Sqrt(16)
Use it to access static methods or properties of a .NET class.
'single'/"double" - Strings
$name = "PowerShell"
Write-Output "Hello, $name!" # Outputs: Hello, PowerShell!
Write-Output 'Hello, $name!' # Outputs: Hello, $name!
Use single quote
to use the strings as is, and double quote
to interpolate strings.
, - Array Separator
$array = 1, 2, 3
$array = $array, 4, 5 # Outputs: 1, 2, 3, 4, 5
Use the comma separator to combine multiple elements.
.. - Range Operator
1..5 # Outputs: 1 2 3 4 5
# creates 1.txt, 2.txt.... 5.txt in the given path
1..5 | % { New-Item -Path "/my/path/$_.txt" -ItemType File }
Use it to create ranges for loops or data sets.
# - Comment
# This is a comment
Write-Output "Hello, World!"
Use it to add comments in code.
> / >> - Redirection
Get-Process > /path/to/processes.txt
Use it to save output to file.
-
>
overwrites the file. -
>>
appends to existing file.
| - Pipeline
Get-Process | Sort-Object CPU
Use it to build data-processing chains.
These shorthand notations can make your PowerShell scripts cleaner and more efficient. Keep this guide handy for quick reference, and practice incorporating these shortcuts into your daily PowerShell usage.
Hope this helps! π