Run a command and get output as object in PowerShell
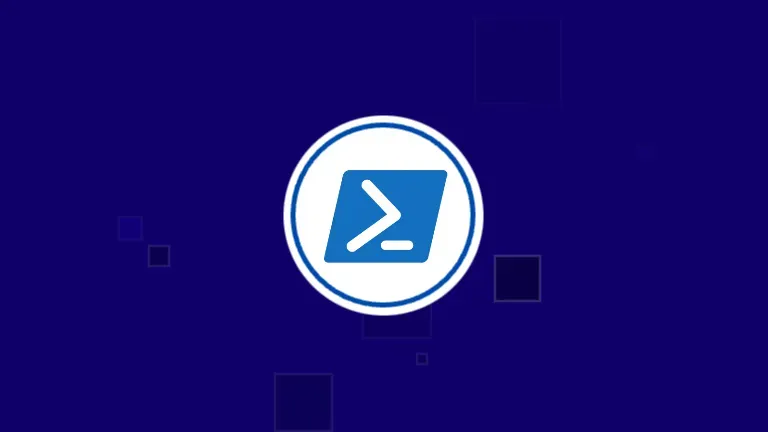
Sometimes it so happens that you have to run a generic Windows command and parse the output in PowerShell. Say you want to execute a command called dsregcmd
. This command gives you
loads of information about the machine you are running it on;
Let's view the normal output of this command in PowerShell.
PS C:> dsregcmd /status
+----------------------------------------------------------------------+
| Device State |
+----------------------------------------------------------------------+
AzureAdJoined : YES
EnterpriseJoined : NO
DomainJoined : NO
Virtual Desktop : NOT SET
Device Name : XXXXX-XXWMY
+----------------------------------------------------------------------+
| Device Details |
+----------------------------------------------------------------------+
DeviceId : a1f5413b-XXXX-XXXX-XXXX-a35c9b227323
Thumbprint : 0FE816B9EAFD3976B85EXXXXXXXXXXXXXXX
...
...
+----------------------------------------------------------------------+
| Tenant Details |
+----------------------------------------------------------------------+
TenantName : Labs
TenantId : d11b204f-XXXX-XXXX-XXXX-15ad85a7675b
...
...
+----------------------------------------------------------------------+
| User State |
+----------------------------------------------------------------------+
NgcSet : NO
WorkplaceJoined : NO
WamDefaultSet : YES
...
...
+----------------------------------------------------------------------+
| SSO State |
+----------------------------------------------------------------------+
AzureAdPrt : YES
AzureAdPrtUpdateTime : 2024-05-28 05:01:57.000 UTC
AzureAdPrtExpiryTime : 2024-06-11 05:01:56.000 UTC
...
...
+----------------------------------------------------------------------+
| Diagnostic Data |
+----------------------------------------------------------------------+
AadRecoveryEnabled : NO
...
...
+----------------------------------------------------------------------+
| IE Proxy Config for Current User |
+----------------------------------------------------------------------+
Auto Detect Settings : YES
Auto-Configuration URL :
Proxy Server List :
Proxy Bypass List :
+----------------------------------------------------------------------+
| WinHttp Default Proxy Config |
+----------------------------------------------------------------------+
Access Type : DIRECT
+----------------------------------------------------------------------+
| Ngc Prerequisite Check |
+----------------------------------------------------------------------+
IsDeviceJoined : YES
IsUserAzureAD : YES
PolicyEnabled : YES
...
...
Step 1: Use the Where-Object or ?
The following is much cleaner:
PS C:> dsregcmd /status | ? { $_ -match ' : ' }
AzureAdJoined : YES
EnterpriseJoined : NO
DomainJoined : NO
Virtual Desktop : NOT SET
...
...
Step 2: Use the Foreach-Object or %
The following clears all the spaces around the text:
PS C:> dsregcmd /status | ? { $_ -match ' : ' } | % { $_.Trim() }
AzureAdJoined : YES
EnterpriseJoined : NO
DomainJoined : NO
Virtual Desktop : NOT SET
...
...
Step 3: ConvertFrom-String to Object
Enough cleaning, let's convert it to object now:
PS C:> dsregcmd /status | ? { $_ -match ' : ' } | % { $_.Trim() } | ConvertFrom-String -PropertyNames 'Name','Value' -Delimiter ' : '
Name Value
---- -----
AzureAdJoined YES
EnterpriseJoined NO
DomainJoined NO
Virtual Desktop NOT SET
Almost done!
Step 4: Save the following function in your PowerShell file
function Get-DeviceInfo([string]$propertyName="all") {
# Run the dsregcmd /status command and process the output
$deviceInfoOutput = dsregcmd /status | Where-Object { $_ -match ' : ' } | % { $_.Trim() } | ConvertFrom-String -PropertyNames 'Name','Value' -Delimiter ' : '
# Convert the resulting array of objects into a hashtable for easier access
$deviceInfo = @{}
$deviceInfoOutput | % { $deviceInfo[$_.Name] = $_.Value }
# Return any property
if($propertyName -eq "all") {
return $deviceInfo
} else {
return $deviceInfo[$propertyName]
}
}
Use it like:
PS C:> Get-DeviceInfo all
Name Value
---- -----
AzureAdJoined YES
EnterpriseJoined NO
DomainJoined NO
Virtual Desktop NOT SET
...
...
PS C:> Get-DeviceInfo AzureAdJoined
YES
Hope this helps!