Check if current logged in user is an admin using PowerShell
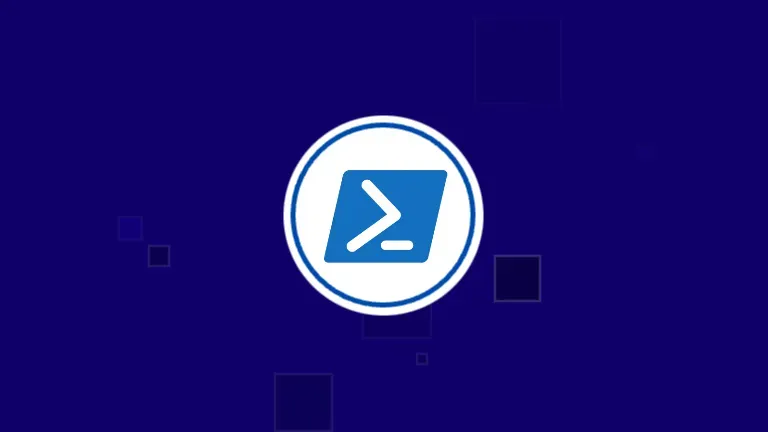
To check if current user is a local administrator, you can use either of the following approaches:
Use the net
command
This command outputs all the users in the administrators
group:
PS C:> net localgroup administrators
Alias name administrators
Comment Administrators have complete and unrestricted access to the computer/domain
Members
-------------------------------------------------------------------------------
user1
LABSDomain Admins
The command completed successfully.
Use the same command with PowerShell
# Get the current logged on user
$currentUser = whoami
# Get the list of users in the Administrators group
$adminGroupMembers = net localgroup administrators
# Check if the current user is part of the Administrators group
if ($adminGroupMembers -contains $currentUser) {
Write-Output "The current user is a part of the Administrators group."
} else {
Write-Output "The current user is NOT a part of the Administrators group."
}
Use System.Security.Principal
However, there is a more robust method using the .NET framework and PowerShell’s Principal objects. This method directly checks the group membership of the current user:
function IsUserAdmin ([boolean]$ShowAllProperties = $false) {
# Get the current logged on user
$currentUser = [System.Security.Principal.WindowsIdentity]::GetCurrent()
if($ShowAllProperties){
$currentUser | Select-Object *
return
}
# Create a principal object for the current user
$userPrincipal = New-Object System.Security.Principal.WindowsPrincipal($currentUser)
# Check if the user is in the Administrators group
if ($userPrincipal.IsInRole(
[System.Security.Principal.WindowsBuiltInRole]::Administrator)
) {
return $true
} else {
return $false
}
}
# This will show all user information. By default, it is $false
IsUserAdmin $true
# This will return True or False
IsUserAdmin
Hope this helps!