PowerShell Basics: Quick Start Guide to Essential Commands and Concepts
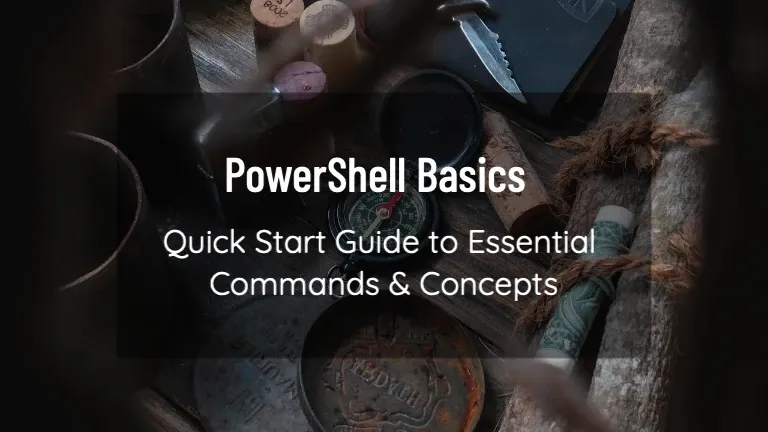
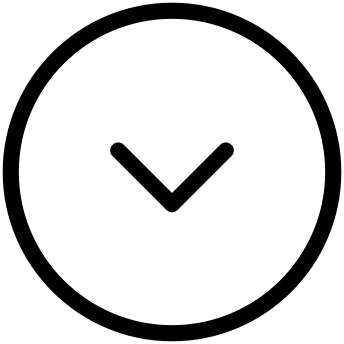
PowerShell is a powerful and flexible scripting language, widely used for automating tasks and managing systems. Whether you’re an IT admin, developer, or just someone looking to quickly pick up PowerShell for your day-to-day tasks, this guide will help you get up to speed in no time. We’ll cover the most essential PowerShell concepts and commands that will enable you to work efficiently.
After you install PowerShell, type $PSVersionTable
and hit enter to Display version related information.
Get-Help Get-Process -Full
2. Working with Files and Directories
PowerShell allows you to easily navigate and manipulate files and directories. The most basic file-related cmdlet is Get-ChildItem
, which lists the contents of a folder (similar to ls
or dir
in Unix-based systems).
Examples:
-
Get-ChildItem -Path C:\
: List files and directories in C:. -
Set-Location -Path C:\
: Change the current directory to C:. -
Copy-Item -Path file.txt -Destination C:\Backup\
: Copy a file to a different location.
If you are on a Mac or Linux:
-
Get-ChildItem -Path /Users/user-name/directory-name/
-
Set-Location -Path /Users/user-name/directory-name/
3. Variables in PowerShell
Variables in PowerShell are prefixed with the $ sign. You can store values, such as strings, integers, or objects, and access them later.
Examples:
$name = "PowerShell"
Write-Output "Hello, $name!"
$myNumber = 42
Write-Output $myNumber
$person = [PSCustomObject]@{
Name = "John Doe"
Age = 30
Occupation = "Developer"
}
Write-Output $person
4. Pipelines: Chaining Cmdlets
One of PowerShell’s powerful features is the ability to use pipelines (`|`) to pass output from one cmdlet to another. This allows for powerful, flexible command combinations.
Get-Process | Where-Object {$_.CPU -gt 100} | Sort-Object CPU -Descending
In the command above, notice how Where-Object
is able to filter out all other output where CPU is less than 100. The output is further piped down to Sort-Object
which sorts the output. Play around with it, it is extremely powerful and fun!
5. Filtering and Sorting Data
PowerShell offers built-in cmdlets for filtering and sorting data. You can filter data based on specific conditions using Where-Object
, and sort the output using Sort-Object
.
Examples:
Get-Process | Where-Object {$_.CPU -gt 100}
Get-Process | Sort-Object CPU -Descending
6. Loops and Conditional Statements
PowerShell allows you to use loops and conditional statements to control the flow of your script.
If-Else Statements:
In this case, PowerShell built in variables that resolve as per the Operating System it is running on...
if ($IsWindows) {
Get-Process
} elseif ($IsMacOS) {
ps aux
} elseif ($IsLinux) {
ps ax
} else {
Write-Output "Platform not supported"
}
ForEach Loop:
$files = Get-ChildItem -Path /user/path
foreach ($file in $files) {
Write-Output $file.Name, $file.Size
}
There is a short hand notation for the same script. Notice how you can avoid using foreach loop using pipes!
$files = Get-ChildItem -Path /user/path
$files | select Name,Size
One more:
$files = Get-ChildItem -Path /user/path
$files | % {
[PSCustomObject]@{
Name = $_.Name.ToUpper()
SizeKB = $_.Size / 1KB
}
}
7. Modules in PowerShell
PowerShell’s functionality can be extended through modules, which are collections of cmdlets and scripts. Modules let you add new features to your environment.
-
Get-InstalledModule
: Shows all modules that are available in your environment, whether installed or just present in the module paths. -
Get-Module -ListAvailable
: Shows only the modules installed through PowerShellGet
You can install modules for various products like so:
Install-Module -Name Az.Websites
To remove modules, use:
Uninstall-Module -Name Az.Websites
Tip
8. Remote Management
Execute commands on remote systems:
Enter-PSSession -ComputerName Server01
Invoke-Command -ComputerName Server01 -ScriptBlock { Get-Service }
Note: To enable remote management, run the following command on the target system:
Enable-PSRemoting -Force
9. Scripts
To create and run scripts, simply save a text file with .ps1
extension.
#script.ps1
Write-Output "Hello, PowerShell! I have your power!!!"
You might have to run the following on your server to allow execution:
Set-ExecutionPolicy -Scope CurrentUser -ExecutionPolicy RemoteSigned
10. Error Handling
To handle errors gracefully, you can use the try catch blocks:
try {
Get-Item "/path/file/does/not/exist" -ErrorAction Stop
} catch {
Write-Output "Error: $_"
}
Notice the usage of -ErrorAction Stop. By default, there are quite a few errors that are non-terminating errors. The Get-Item
cmdlet by default throws a non-terminating error when it fails to find a file. This means the script continues execution even though the error occurs. To ensure that try/catch works as expected, it has been explicitly written to stop on error.
I hope this helps! Stay tuned for more. 👋