Insert and extract a binary file in C# assembly at run time
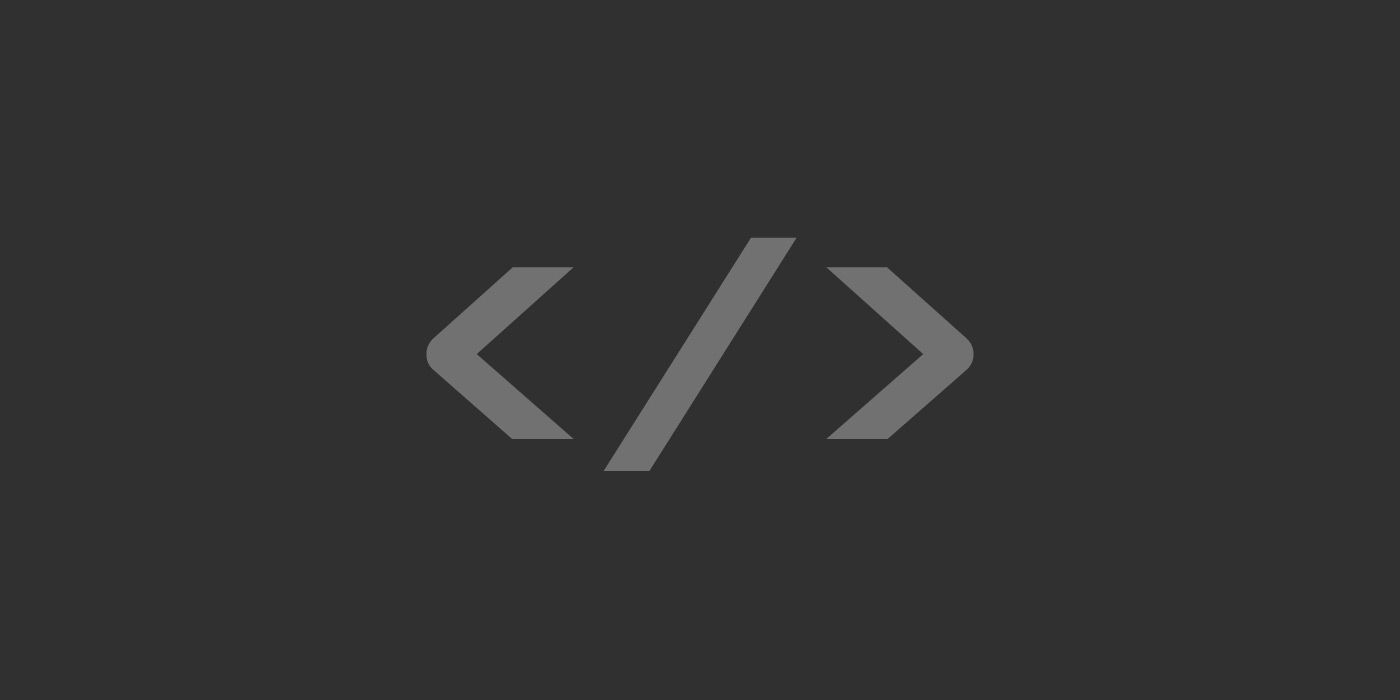
Problem
There is a binary file (I’ll use an Excel file) that you want to embed in any C# assembly. Later on, at runtime, you want to extract the file from the EXE and continue working on it. In this post, I will share the code and describe how you can do that. You can use the same technique for any other kind of binary file as well.
Solution
First up, the reason why you may choose to embed a resource directly into your EXE is to ensure that no one tampers your file on which your code is based. This post doesn’t talk about handling an Excel file. It mainly focuses on two parts… embedding any file directly in your EXE, and then extract it at runtime and execute it.
-
Create a new Windows Forms Project
-
Add an Existing file (I’ve added a file called File.xlsx) in the project
-
Right click on File.xlsx and select Properties
-
Change the Build Action –> Embedded Resource
-
Switch to the form where you want to extract and use this resource
-
Add the following to the top of the code behind...
using System.Reflection;
using System.IO;
- Add the following methods and call ExtractFromAssembly method
private void ExtractFromAssembly()
{
string strPath = Application.LocalUserAppDataPath + "OutputFile.xlsx";
if (File.Exists(strPath)) File.Delete(strPath);
Assembly assembly = Assembly.GetExecutingAssembly();
//In the next line you should provide NameSpace.FileName.Extension that you have embedded
var input = assembly.GetManifestResourceStream("Sample.File.xlsx");
var output = File.Open(strPath, FileMode.CreateNew);
CopyStream(input, output);
input.Dispose();
output.Dispose();
System.Diagnostics.Process.Start(strPath);
}
private void CopyStream(Stream input, Stream output)
{
byte[] buffer = new byte[32768];
while (true)
{
int read = input.Read(buffer, 0, buffer.Length);
if (read <= 0)
return;
output.Write(buffer, 0, read);
}
}
Hope it helps.